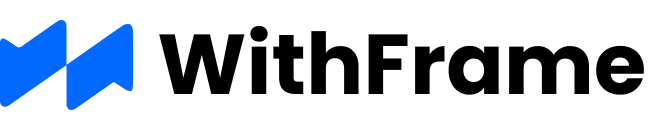
Button Groups
Button groups are a powerful tool for organizing content across multiple screens. With pre-built code components, you can easily create toggle button groups that allow users to switch between different screens and access the information they need quickly and easily. For any mobile applications button groups are an essential component that can help you create a user-friendly and intuitive interface.
Our pre-built code components for button groups are designed to flexible and very easy to use. With a few lines of code, you can customize the look and feel of your button group, choose the number of buttons you need, and add text or icons to each button.
import React from 'react';
import {
StyleSheet,
View,
Text,
TouchableWithoutFeedback,
SafeAreaView,
} from 'react-native';
const tabs = [{ name: 'My Account' }, { name: 'Company' }, { name: 'Team' }];
export default function Example() {
const [value, setValue] = React.useState(0);
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
{tabs.map((item, index) => {
const isActive = index === value;
return (
<View key={item.name} style={{ flex: 1 }}>
<TouchableWithoutFeedback
onPress={() => {
setValue(index);
}}>
<View
style={[
styles.item,
isActive && { backgroundColor: '#e0e7ff' },
]}>
<Text style={[styles.text, isActive && { color: '#4338ca' }]}>
{item.name}
</Text>
</View>
</TouchableWithoutFeedback>
</View>
);
})}
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flexDirection: 'row',
backgroundColor: 'white',
paddingVertical: 24,
paddingHorizontal: 12,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
item: {
justifyContent: 'center',
alignItems: 'center',
paddingVertical: 10,
backgroundColor: 'transparent',
borderRadius: 6,
},
text: {
fontSize: 13,
fontWeight: '600',
color: '#6b7280',
},
});
import React from 'react';
import {
StyleSheet,
View,
Text,
TouchableWithoutFeedback,
SafeAreaView,
} from 'react-native';
const tabs = [{ name: 'My Account' }, { name: 'Company' }, { name: 'Team' }];
export default function Example() {
const [value, setValue] = React.useState(0);
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
{tabs.map((item, index) => {
const isActive = index === value;
return (
<View key={item.name} style={{ flex: 1 }}>
<TouchableWithoutFeedback
onPress={() => {
setValue(index);
}}>
<View
style={[
styles.item,
isActive && { backgroundColor: '#e5e7eb' },
]}>
<Text style={[styles.text, isActive && { color: '#374151' }]}>
{item.name}
</Text>
</View>
</TouchableWithoutFeedback>
</View>
);
})}
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flexDirection: 'row',
backgroundColor: 'white',
paddingVertical: 24,
paddingHorizontal: 12,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
item: {
justifyContent: 'center',
alignItems: 'center',
paddingVertical: 10,
backgroundColor: 'transparent',
borderRadius: 6,
},
text: {
fontSize: 13,
fontWeight: '600',
color: '#6b7280',
},
});
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;