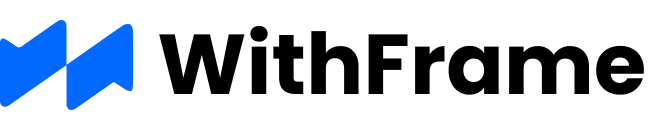
Profile Cards
Our collection of profile cards are designed to be customizable and easy to use, making it simple for you to create a professional-looking profile page in no time.
Each component is created with clean, modern design principles in mind, ensuring that your profile pages not only function well but also look great. Whether you’re building a social network, an e-commerce app, or any other type of application that requires a profile page, our pre-built components are the perfect solution.
-
Left-Aligned Avatar and Two-Line Text
-
Centered Avatar, Two-Line Text, and Button
-
Left-Aligned Avatar, Two-Line Text, and Button
-
Centered Avatar and Two-Line Text with Action Icon
-
Left-Aligned Avatar, Two-Line Text, Profile Description, and Tags
import React from 'react';
import { StyleSheet, SafeAreaView, View, Image, Text } from 'react-native';
export default function Example() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#f6f6f6' }}>
<View style={styles.container}>
<View style={styles.profile}>
<Image
alt=""
source={{
uri: 'https://images.unsplash.com/photo-1599566150163-29194dcaad36?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=facearea&facepad=2.5&w=256&h=256&q=80',
}}
style={styles.profileAvatar} />
<View>
<Text style={styles.profileName}>Roland Gilbert</Text>
<Text style={styles.profileHandle}>
[email protected]
</Text>
</View>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
paddingVertical: 48,
paddingHorizontal: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
/** Profile */
profile: {
padding: 12,
backgroundColor: '#fff',
borderRadius: 12,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'flex-start',
},
profileAvatar: {
width: 60,
height: 60,
borderRadius: 9999,
marginRight: 12,
},
profileName: {
fontSize: 18,
fontWeight: '600',
color: '#292929',
},
profileHandle: {
marginTop: 2,
fontSize: 16,
fontWeight: '400',
color: '#858585',
},
});
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;