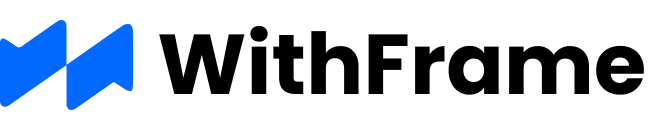
Sign In Forms
Our collection of sign-in form examples goes beyond the traditional email and password login, offering you a diverse range of options that can be customized to fit your unique needs. From social media logins to biometric authentication our pre-built components will allow you to create an exclusive sign-in experience that is not only secure but also user friendly.
import React, { useState } from 'react';
import {
StyleSheet,
SafeAreaView,
View,
Text,
TouchableOpacity,
TextInput,
} from 'react-native';
export default function Example() {
const [form, setForm] = useState({
email: '',
password: '',
});
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
<View style={styles.header}>
<Text style={styles.title}>Welcome back!</Text>
<Text style={styles.subtitle}>Sign in to your account</Text>
</View>
<View style={styles.form}>
<View style={styles.input}>
<Text style={styles.inputLabel}>Email address</Text>
<TextInput
autoCapitalize="none"
autoCorrect={false}
clearButtonMode="while-editing"
keyboardType="email-address"
onChangeText={email => setForm({ ...form, email })}
placeholder="[email protected]"
placeholderTextColor="#6b7280"
style={styles.inputControl}
value={form.email} />
</View>
<View style={styles.input}>
<Text style={styles.inputLabel}>Password</Text>
<TextInput
autoCorrect={false}
clearButtonMode="while-editing"
onChangeText={password => setForm({ ...form, password })}
placeholder="********"
placeholderTextColor="#6b7280"
style={styles.inputControl}
secureTextEntry={true}
value={form.password} />
</View>
<View style={styles.formAction}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btn}>
<Text style={styles.btnText}>Sign in</Text>
</View>
</TouchableOpacity>
</View>
<TouchableOpacity
onPress={() => {
// handle link
}}>
<Text style={styles.formFooter}>
Don't have an account?{' '}
<Text style={{ textDecorationLine: 'underline' }}>Sign up</Text>
</Text>
</TouchableOpacity>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
header: {
marginVertical: 36,
},
title: {
fontSize: 32,
fontWeight: 'bold',
color: '#1d1d1d',
marginBottom: 6,
textAlign: 'center',
},
subtitle: {
fontSize: 15,
fontWeight: '500',
color: '#929292',
textAlign: 'center',
},
/** Form */
form: {
marginBottom: 24,
},
formAction: {
marginVertical: 24,
},
formFooter: {
fontSize: 15,
fontWeight: '500',
color: '#222',
textAlign: 'center',
},
/** Input */
input: {
marginBottom: 16,
},
inputLabel: {
fontSize: 17,
fontWeight: '600',
color: '#222',
marginBottom: 8,
},
inputControl: {
height: 44,
backgroundColor: '#f1f5f9',
paddingHorizontal: 16,
borderRadius: 12,
fontSize: 15,
fontWeight: '500',
color: '#222',
},
/** Button */
btn: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#007aff',
borderColor: '#007aff',
},
btnText: {
fontSize: 17,
lineHeight: 24,
fontWeight: '600',
color: '#fff',
},
});
Part of the Simple Onboarding Flow (5 Screens).
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
Part of the Modern Onboarding Flow (7 Screens).
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
Part of the Simple Inline Onboarding Flow (6 Screens).
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;