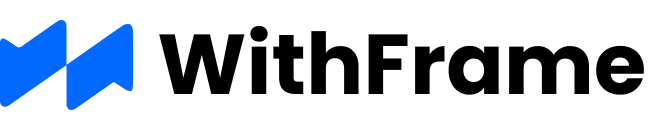
Counters
Whether you are building an e-commerce app, an order cart, or a pricing screen, quantity counters are a great way to engage your users and encourage them to take action.
With our code components, you can easily create visually appealing and functional quantity counters that will enhance the user experience on your app.
import React from 'react';
import {
StyleSheet,
SafeAreaView,
View,
TouchableOpacity,
Text,
} from 'react-native';
export default function Example() {
const [value, setValue] = React.useState(0);
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
<View style={styles.counter}>
<TouchableOpacity
disabled={value <= 0}
onPress={() => {
setValue(Math.max(value - 1, 0));
}}
style={styles.counterAction}>
<Text style={styles.counterActionText}>-</Text>
</TouchableOpacity>
<Text style={styles.counterValue}>{value}</Text>
<TouchableOpacity
onPress={() => {
setValue(value + 1);
}}
style={styles.counterAction}>
<Text style={styles.counterActionText}>+</Text>
</TouchableOpacity>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
/** Counter */
counter: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
},
counterAction: {
width: 34,
height: 34,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderColor: '#98d0ca',
borderWidth: 2,
borderRadius: 9999,
},
counterActionText: {
fontSize: 20,
lineHeight: 20,
fontWeight: '600',
color: '#98d0ca',
},
counterValue: {
minWidth: 44,
fontSize: 19,
fontWeight: '600',
color: '#1d1d1d',
textAlign: 'center',
paddingHorizontal: 8,
},
});
import React from 'react';
import {
StyleSheet,
SafeAreaView,
View,
TouchableOpacity,
Text,
} from 'react-native';
export default function Example() {
const [value, setValue] = React.useState(0);
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
<View style={styles.counter}>
<TouchableOpacity
disabled={value <= 0}
onPress={() => {
setValue(Math.max(value - 1, 0));
}}
style={styles.counterAction}>
<Text style={styles.counterActionText}>-</Text>
</TouchableOpacity>
<Text style={styles.counterValue}>{value}</Text>
<TouchableOpacity
onPress={() => {
setValue(value + 1);
}}
style={styles.counterAction}>
<Text style={styles.counterActionText}>+</Text>
</TouchableOpacity>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
/** Counter */
counter: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
alignSelf: 'center',
borderWidth: 1,
borderColor: '#e1e1e1',
borderStyle: 'solid',
borderRadius: 8,
},
counterAction: {
width: 46,
height: 34,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
},
counterActionText: {
fontSize: 20,
lineHeight: 20,
fontWeight: '500',
color: '#000',
},
counterValue: {
minWidth: 34,
fontSize: 14,
fontWeight: '500',
color: '#101010',
textAlign: 'center',
paddingHorizontal: 8,
},
});
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;