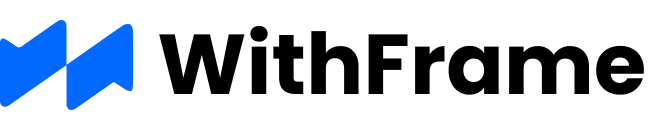
Settings Forms
Our collection of settings screens are designed to make it easy for your users to change their settings in a hassle-free way, without compromising on aesthetics or functionality.
Whether you need a simple settings screen with basic options, or a complex one with multiple categories and sub-categories, our collection has got you covered. Our pre-built components are designed to seamlessly integrate with your existing codebase, allowing you to save time and focus on other critical aspects of your application.
-
Simple Settings Form
-
Full-Width Settings Form
-
Full-Width Settings Form with Tabs
-
Modern Settings Form with Toggles
Part of the Simple Profile Setup Flow (8 Screens)
.
import React, { useState } from 'react';
import {
StyleSheet,
SafeAreaView,
ScrollView,
View,
Text,
TouchableOpacity,
Switch,
Image,
} from 'react-native';
import FeatherIcon from 'react-native-vector-icons/Feather''@expo/vector-icons/Feather';
export default function Example() {
const [form, setForm] = useState({
emailNotifications: true,
pushNotifications: false,
});
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#f8f8f8' }}>
<View style={styles.header}>
<View style={styles.headerAction}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<FeatherIcon
color="#000"
name="arrow-left"
size={24} />
</TouchableOpacity>
</View>
<Text numberOfLines={1} style={styles.headerTitle}>
Settings
</Text>
<View style={[styles.headerAction, { alignItems: 'flex-end' }]}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<FeatherIcon
color="#000"
name="more-vertical"
size={24} />
</TouchableOpacity>
</View>
</View>
<ScrollView contentContainerStyle={styles.content}>
<View style={[styles.section, { paddingTop: 4 }]}>
<Text style={styles.sectionTitle}>Account</Text>
<View style={styles.sectionBody}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}
style={styles.profile}>
<Image
alt=""
source={{
uri: 'https://images.unsplash.com/photo-1633332755192-727a05c4013d?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=facearea&facepad=2.5&w=256&h=256&q=80',
}}
style={styles.profileAvatar} />
<View style={styles.profileBody}>
<Text style={styles.profileName}>John Doe</Text>
<Text style={styles.profileHandle}>[email protected]</Text>
</View>
<FeatherIcon
color="#bcbcbc"
name="chevron-right"
size={22} />
</TouchableOpacity>
</View>
</View>
<View style={styles.section}>
<Text style={styles.sectionTitle}>Preferences</Text>
<View style={styles.sectionBody}>
<View style={[styles.rowWrapper, styles.rowFirst]}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}
style={styles.row}>
<Text style={styles.rowLabel}>Language</Text>
<View style={styles.rowSpacer} />
<Text style={styles.rowValue}>English</Text>
<FeatherIcon
color="#bcbcbc"
name="chevron-right"
size={19} />
</TouchableOpacity>
</View>
<View style={styles.rowWrapper}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}
style={styles.row}>
<Text style={styles.rowLabel}>Location</Text>
<View style={styles.rowSpacer} />
<Text style={styles.rowValue}>Los Angeles, CA</Text>
<FeatherIcon
color="#bcbcbc"
name="chevron-right"
size={19} />
</TouchableOpacity>
</View>
<View style={styles.rowWrapper}>
<View style={styles.row}>
<Text style={styles.rowLabel}>Email Notifications</Text>
<View style={styles.rowSpacer} />
<Switch
onValueChange={emailNotifications =>
setForm({ ...form, emailNotifications })
}
style={{ transform: [{ scaleX: 0.95 }, { scaleY: 0.95 }] }}
value={form.emailNotifications} />
</View>
</View>
<View style={[styles.rowWrapper, styles.rowLast]}>
<View style={styles.row}>
<Text style={styles.rowLabel}>Push Notifications</Text>
<View style={styles.rowSpacer} />
<Switch
onValueChange={pushNotifications =>
setForm({ ...form, pushNotifications })
}
style={{ transform: [{ scaleX: 0.95 }, { scaleY: 0.95 }] }}
value={form.pushNotifications} />
</View>
</View>
</View>
</View>
<View style={styles.section}>
<Text style={styles.sectionTitle}>Resources</Text>
<View style={styles.sectionBody}>
<View style={[styles.rowWrapper, styles.rowFirst]}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}
style={styles.row}>
<Text style={styles.rowLabel}>Contact Us</Text>
<View style={styles.rowSpacer} />
<FeatherIcon
color="#bcbcbc"
name="chevron-right"
size={19} />
</TouchableOpacity>
</View>
<View style={styles.rowWrapper}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}
style={styles.row}>
<Text style={styles.rowLabel}>Report Bug</Text>
<View style={styles.rowSpacer} />
<FeatherIcon
color="#bcbcbc"
name="chevron-right"
size={19} />
</TouchableOpacity>
</View>
<View style={styles.rowWrapper}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}
style={styles.row}>
<Text style={styles.rowLabel}>Rate in App Store</Text>
<View style={styles.rowSpacer} />
<FeatherIcon
color="#bcbcbc"
name="chevron-right"
size={19} />
</TouchableOpacity>
</View>
<View style={[styles.rowWrapper, styles.rowLast]}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}
style={styles.row}>
<Text style={styles.rowLabel}>Terms and Privacy</Text>
<View style={styles.rowSpacer} />
<FeatherIcon
color="#bcbcbc"
name="chevron-right"
size={19} />
</TouchableOpacity>
</View>
</View>
</View>
<View style={styles.section}>
<View style={styles.sectionBody}>
<View
style={[
styles.rowWrapper,
styles.rowFirst,
styles.rowLast,
{ alignItems: 'center' },
]}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}
style={styles.row}>
<Text style={[styles.rowLabel, styles.rowLabelLogout]}>
Log Out
</Text>
</TouchableOpacity>
</View>
</View>
</View>
<Text style={styles.contentFooter}>App Version 2.24 #50491</Text>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
/** Header */
header: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'space-between',
width: '100%',
paddingHorizontal: 16,
},
headerAction: {
width: 40,
height: 40,
alignItems: 'flex-start',
justifyContent: 'center',
},
headerTitle: {
fontSize: 19,
fontWeight: '600',
color: '#000',
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
textAlign: 'center',
},
/** Content */
content: {
paddingHorizontal: 16,
},
contentFooter: {
marginTop: 24,
fontSize: 13,
fontWeight: '500',
textAlign: 'center',
color: '#a69f9f',
},
/** Section */
section: {
paddingVertical: 12,
},
sectionTitle: {
margin: 8,
marginLeft: 12,
fontSize: 13,
letterSpacing: 0.33,
fontWeight: '500',
color: '#a69f9f',
textTransform: 'uppercase',
},
sectionBody: {
borderRadius: 12,
shadowColor: '#000',
shadowOffset: {
width: 0,
height: 1,
},
shadowOpacity: 0.2,
shadowRadius: 1.41,
elevation: 2,
},
/** Profile */
profile: {
padding: 12,
backgroundColor: '#fff',
borderRadius: 12,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'flex-start',
},
profileAvatar: {
width: 60,
height: 60,
borderRadius: 9999,
marginRight: 12,
},
profileBody: {
marginRight: 'auto',
},
profileName: {
fontSize: 18,
fontWeight: '600',
color: '#292929',
},
profileHandle: {
marginTop: 2,
fontSize: 16,
fontWeight: '400',
color: '#858585',
},
/** Row */
row: {
height: 44,
width: '100%',
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'flex-start',
paddingRight: 12,
},
rowWrapper: {
paddingLeft: 16,
backgroundColor: '#fff',
borderTopWidth: 1,
borderColor: '#f0f0f0',
},
rowFirst: {
borderTopLeftRadius: 12,
borderTopRightRadius: 12,
},
rowLabel: {
fontSize: 16,
letterSpacing: 0.24,
color: '#000',
},
rowSpacer: {
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
rowValue: {
fontSize: 16,
fontWeight: '500',
color: '#ababab',
marginRight: 4,
},
rowLast: {
borderBottomLeftRadius: 12,
borderBottomRightRadius: 12,
},
rowLabelLogout: {
width: '100%',
textAlign: 'center',
fontWeight: '600',
color: '#dc2626',
},
});
Part of the Full-Width Profile Setup Flow (8 Screens)
.
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;