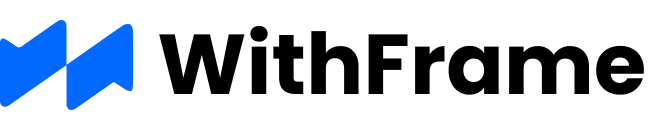
Landing Pages
Our landing page components are designed to help you convert visitors into users, providing you with a range of options whether you’re launching a new product or service, or simply looking to improve your presence.
-
Simple Landing Page
-
Modern Landing Page
-
Social Media Landing Page
-
Landing Page with Photos and Overlay Text
-
Landing Page with Background Image
-
Multi-Screen Landing Page
-
Multi-Screen Dark Mode Landing Page
import React from 'react';
import {
StyleSheet,
View,
Text,
SafeAreaView,
Image,
TouchableOpacity,
} from 'react-native';
export default function Example() {
return (
<SafeAreaView style={styles.container}>
<View style={styles.hero}>
<Image
source={{ uri: 'https://assets.withfra.me/Landing.3.png' }}
style={styles.heroImage}
resizeMode="contain"
/>
</View>
<View style={styles.content}>
<View style={styles.contentHeader}>
<Text style={styles.title}>
Plan your day{'\n'}with{' '}
<View style={styles.appName}>
<Text style={styles.appNameText}>MyApp</Text>
</View>
</Text>
<Text style={styles.text}>
Aliqua ullamco incididunt elit labore consequat ipsum sunt
exercitation aliqua duis nulla et qui fugiat
</Text>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.button}>
<Text style={styles.buttonText}>Let's go</Text>
</View>
</TouchableOpacity>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
},
title: {
fontSize: 28,
fontWeight: '500',
color: '#281b52',
textAlign: 'center',
marginBottom: 12,
lineHeight: 40,
},
text: {
fontSize: 15,
lineHeight: 24,
fontWeight: '400',
color: '#9992a7',
textAlign: 'center',
},
/** Hero */
hero: {
backgroundColor: '#d8dffe',
margin: 12,
borderRadius: 16,
padding: 16,
},
heroImage: {
width: '100%',
height: 400,
},
/** Content */
content: {
flex: 1,
justifyContent: 'space-between',
paddingVertical: 24,
paddingHorizontal: 24,
},
contentHeader: {
paddingHorizontal: 24,
},
appName: {
backgroundColor: '#fff2dd',
transform: [
{
rotate: '-5deg',
},
],
paddingHorizontal: 6,
},
appNameText: {
fontSize: 28,
fontWeight: '700',
color: '#281b52',
},
/** Button */
button: {
backgroundColor: '#56409e',
paddingVertical: 12,
paddingHorizontal: 14,
alignItems: 'center',
justifyContent: 'center',
borderRadius: 12,
},
buttonText: {
fontSize: 15,
fontWeight: '500',
color: '#fff',
},
});
Part of the Modern Onboarding Flow (16 Screens)
.
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;