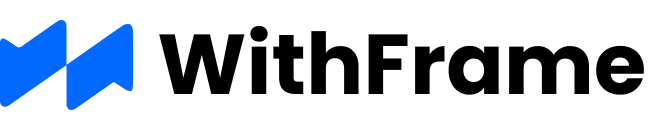
Home Screens
Home screens are the gateway to your app, and they are often the first impression that users get when they interact with your mobile application.
Our pre-built code components for home screens are designed to be highly customizable and easy to use.
- Free
Simple Home Screen
-
Food Delivery Home Screen
-
Clothing Store Home Screen
-
Dark Mode Home Screen
-
Home Screen with Search Field
-
Home Screen with Actions
-
Home Screen with Banner
-
Home Screen with Balance
import React from 'react';
import {
StyleSheet,
SafeAreaView,
View,
Text,
TouchableOpacity,
TextInput,
} from 'react-native';
import FeatherIcon from 'react-native-vector-icons/Feather''@expo/vector-icons/Feather';
export default function Example() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
<View>
<View style={styles.actionWrapper}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}
style={{ marginRight: 'auto' }}>
<View style={styles.action}>
<FeatherIcon
color="#6a99e3"
name="menu"
size={22} />
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.action}>
<FeatherIcon
color="#6a99e3"
name="bell"
size={22} />
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.action}>
<FeatherIcon
color="#6a99e3"
name="user"
size={22} />
</View>
</TouchableOpacity>
</View>
<Text style={styles.title}>Online Orders</Text>
<View style={styles.search}>
<View style={styles.searchInput}>
<View style={styles.inputWrapper}>
<TextInput
placeholder="Enter tracking code"
placeholderTextColor="#9eadba"
style={styles.input} />
<View style={styles.inputIcon}>
<FeatherIcon
color="#9eadba"
name="box"
size={16} />
</View>
</View>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btn}>
<Text style={styles.btnText}>Submit</Text>
</View>
</TouchableOpacity>
</View>
</View>
<View style={styles.placeholder}>
<View style={styles.placeholderInset}>
{/* Replace with your content */}
</View>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
title: {
fontSize: 27,
fontWeight: '700',
color: '#222',
marginTop: 24,
marginBottom: 16,
},
/** Action */
action: {
width: 48,
height: 48,
borderRadius: 12,
marginHorizontal: 8,
backgroundColor: '#e8f0f9',
alignItems: 'center',
justifyContent: 'center',
},
actionWrapper: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'flex-start',
marginHorizontal: -8,
},
/** Search */
search: {
flexDirection: 'row',
alignItems: 'center',
},
searchInput: {
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
marginRight: 12,
},
/** Input */
input: {
height: 44,
backgroundColor: '#f0f6fb',
paddingLeft: 44,
paddingRight: 24,
borderRadius: 12,
fontSize: 15,
fontWeight: '500',
color: '#222',
},
inputWrapper: {
position: 'relative',
width: '100%',
},
inputIcon: {
position: 'absolute',
width: 44,
height: 44,
top: 0,
left: 0,
bottom: 0,
alignItems: 'center',
justifyContent: 'center',
},
/** Button */
btn: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#222',
borderColor: '#222',
},
btnText: {
fontSize: 17,
lineHeight: 24,
fontWeight: '600',
color: '#fff',
},
/** Placeholder */
placeholder: {
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
height: 400,
marginTop: 24,
padding: 0,
backgroundColor: 'transparent',
},
placeholderInset: {
borderWidth: 4,
borderColor: '#e5e7eb',
borderStyle: 'dashed',
borderRadius: 9,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
});
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;