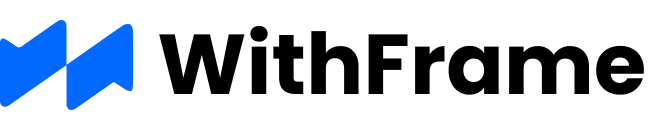
Buttons
Buttons are one of the most essential components in user interface design. With pre-built code components for buttons, you can easily create visually appealing and responsive buttons that enhance the user experience and improve engagement.
Our pre-built code components for buttons are designed to be highly customizable and easy to use. With just a few lines of code, you can create buttons in a variety of shapes, sizes, and colors, and add custom text and icons to them. Our components are also optimized for speed and performance, so your buttons will load quickly and efficiently, even on slow connections.
import React from 'react';
import {
StyleSheet,
View,
SafeAreaView,
TouchableOpacity,
Text,
} from 'react-native';
export default function Example() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
<View style={styles.buttons}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnXS}>
<Text style={styles.btnXSText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnSM}>
<Text style={styles.btnSMText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnMD}>
<Text style={styles.btnMDText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnLG}>
<Text style={styles.btnLGText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnXL}>
<Text style={styles.btnXLText}>Custom Button</Text>
</View>
</TouchableOpacity>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
buttons: {
height: 300,
alignItems: 'center',
justifyContent: 'space-between',
},
btnXS: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 6,
paddingHorizontal: 14,
borderWidth: 1,
backgroundColor: '#0569FF',
borderColor: '#0569FF',
},
btnXSText: {
fontSize: 13,
lineHeight: 18,
fontWeight: '600',
color: '#fff',
},
btnSM: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#0569FF',
borderColor: '#0569FF',
},
btnSMText: {
fontSize: 14,
lineHeight: 20,
fontWeight: '600',
color: '#fff',
},
btnMD: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#0569FF',
borderColor: '#0569FF',
},
btnMDText: {
fontSize: 17,
lineHeight: 24,
fontWeight: '600',
color: '#fff',
},
btnLG: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 10,
paddingHorizontal: 20,
borderWidth: 1,
backgroundColor: '#0569FF',
borderColor: '#0569FF',
},
btnLGText: {
fontSize: 18,
lineHeight: 26,
fontWeight: '600',
color: '#fff',
},
btnXL: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 16,
paddingHorizontal: 24,
borderWidth: 1,
backgroundColor: '#0569FF',
borderColor: '#0569FF',
},
btnXLText: {
fontSize: 20,
lineHeight: 28,
fontWeight: '600',
color: '#fff',
},
});
import React from 'react';
import {
StyleSheet,
View,
SafeAreaView,
TouchableOpacity,
Text,
} from 'react-native';
export default function Example() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
<View style={styles.buttons}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnXS}>
<Text style={styles.btnXSText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnSM}>
<Text style={styles.btnSMText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnMD}>
<Text style={styles.btnMDText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnLG}>
<Text style={styles.btnLGText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnXL}>
<Text style={styles.btnXLText}>Custom Button</Text>
</View>
</TouchableOpacity>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
buttons: {
height: 300,
alignItems: 'center',
justifyContent: 'space-between',
},
btnXS: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 6,
paddingHorizontal: 14,
borderWidth: 1,
backgroundColor: '#efefef',
borderColor: '#efefef',
},
btnXSText: {
fontSize: 13,
lineHeight: 18,
fontWeight: '600',
color: '#0d57b2',
},
btnSM: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#efefef',
borderColor: '#efefef',
},
btnSMText: {
fontSize: 14,
lineHeight: 20,
fontWeight: '600',
color: '#0d57b2',
},
btnMD: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#efefef',
borderColor: '#efefef',
},
btnMDText: {
fontSize: 17,
lineHeight: 24,
fontWeight: '600',
color: '#0d57b2',
},
btnLG: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 10,
paddingHorizontal: 20,
borderWidth: 1,
backgroundColor: '#efefef',
borderColor: '#efefef',
},
btnLGText: {
fontSize: 18,
lineHeight: 26,
fontWeight: '600',
color: '#0d57b2',
},
btnXL: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 16,
paddingHorizontal: 24,
borderWidth: 1,
backgroundColor: '#efefef',
borderColor: '#efefef',
},
btnXLText: {
fontSize: 20,
lineHeight: 28,
fontWeight: '600',
color: '#0d57b2',
},
});
import React from 'react';
import {
StyleSheet,
View,
SafeAreaView,
TouchableOpacity,
Text,
} from 'react-native';
export default function Example() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
<View style={styles.buttons}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnXS}>
<Text style={styles.btnXSText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnSM}>
<Text style={styles.btnSMText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnMD}>
<Text style={styles.btnMDText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnLG}>
<Text style={styles.btnLGText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnXL}>
<Text style={styles.btnXLText}>Custom Button</Text>
</View>
</TouchableOpacity>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
buttons: {
height: 300,
alignItems: 'center',
justifyContent: 'space-between',
},
btnXS: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 6,
paddingHorizontal: 14,
borderWidth: 1,
backgroundColor: '#fff',
borderColor: '#d1d5db',
},
btnXSText: {
fontSize: 13,
lineHeight: 18,
fontWeight: '600',
color: '#374151',
},
btnSM: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#fff',
borderColor: '#d1d5db',
},
btnSMText: {
fontSize: 14,
lineHeight: 20,
fontWeight: '600',
color: '#374151',
},
btnMD: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#fff',
borderColor: '#d1d5db',
},
btnMDText: {
fontSize: 17,
lineHeight: 24,
fontWeight: '600',
color: '#374151',
},
btnLG: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 10,
paddingHorizontal: 20,
borderWidth: 1,
backgroundColor: '#fff',
borderColor: '#d1d5db',
},
btnLGText: {
fontSize: 18,
lineHeight: 26,
fontWeight: '600',
color: '#374151',
},
btnXL: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 16,
paddingHorizontal: 24,
borderWidth: 1,
backgroundColor: '#fff',
borderColor: '#d1d5db',
},
btnXLText: {
fontSize: 20,
lineHeight: 28,
fontWeight: '600',
color: '#374151',
},
});
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;