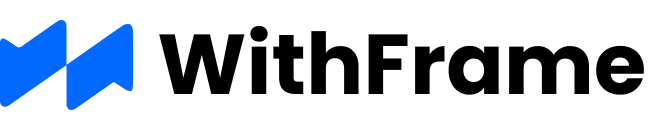
Alerts
Our pre-built alert components are designed to be customizable and easy to use, built using the latest web technologies and can be integrated into your existing React Native codebase.
With our pre-built alerts components, you can create alerts for a wide range of scenarios, including error messages, success notifications, and confirmation dialogs. You can also customize the appearance and behavior of your alerts to match the design and functionality of your react native application.
-
Full Screen Alert with Image and Actions
-
Alert: Top Banner with Dismiss Button
-
Message Request Alert in Chat Screen
-
Dark Mode Alert in Chat Screen
-
Alert: Full Screen with Actions
-
Half-Screen Congratulations Alert
import React from 'react';
import {
StyleSheet,
SafeAreaView,
View,
Image,
Text,
TouchableOpacity,
} from 'react-native';
export default function Example() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
<View style={styles.alert}>
<View style={styles.alertContent}>
<Image
alt=""
style={styles.alertAvatar}
source={{
uri: 'https://images.unsplash.com/photo-1633332755192-727a05c4013d?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=facearea&facepad=2.5&w=256&h=256&q=80',
}} />
<Text style={styles.alertTitle}>
Log out of
{'\n'}
@MarkSimmons
</Text>
<Text style={styles.alertMessage}>
Are you sure you would like to log out of this account? You will
need your password to log back in.
</Text>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btn}>
<Text style={styles.btnText}>Yes, log me out</Text>
</View>
</TouchableOpacity>
<View style={{ marginTop: 8 }}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnSecondary}>
<Text style={styles.btnSecondaryText}>Cancel</Text>
</View>
</TouchableOpacity>
</View>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
/** Alert */
alert: {
position: 'relative',
flexDirection: 'column',
alignItems: 'stretch',
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
paddingTop: 80,
},
alertContent: {
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
alertAvatar: {
width: 160,
height: 160,
borderRadius: 9999,
alignSelf: 'center',
marginBottom: 24,
},
alertTitle: {
marginBottom: 16,
fontSize: 34,
lineHeight: 44,
fontWeight: '700',
color: '#000',
textAlign: 'center',
},
alertMessage: {
marginBottom: 24,
textAlign: 'center',
fontSize: 16,
lineHeight: 22,
fontWeight: '500',
color: '#9a9a9a',
},
/** Button */
btn: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#f75249',
borderColor: '#f75249',
},
btnText: {
fontSize: 17,
lineHeight: 24,
fontWeight: '600',
color: '#fff',
},
btnSecondary: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: 'transparent',
borderColor: 'transparent',
},
btnSecondaryText: {
fontSize: 17,
lineHeight: 24,
fontWeight: '600',
color: '#f75249',
},
});
import React from 'react';
import {
StyleSheet,
View,
SafeAreaView,
Text,
TouchableOpacity,
} from 'react-native';
import FeatherIcon from 'react-native-vector-icons/Feather''@expo/vector-icons/Feather';
export default function Example() {
return (
<SafeAreaView>
<View style={styles.container}>
<View style={styles.alert}>
<View
style={[
styles.alertIcon,
{ backgroundColor: '#14b073', borderColor: '#14b073' },
]}>
<FeatherIcon
color="#fff"
name="credit-card"
size={30} />
</View>
<View style={styles.alertBody}>
<Text style={styles.alertTitle}>Credit card updated!</Text>
<Text style={styles.alertMessage}>
Lorem nisi enim laboris quis elit. nisi enim laboris quis elit.
</Text>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.alertClose}>
<FeatherIcon color="#9a9a9a" name="x" size={24} />
</View>
</TouchableOpacity>
</View>
<View style={styles.alert}>
<View
style={[
styles.alertIcon,
{ backgroundColor: '#fa5855', borderColor: '#fa5855' },
]}>
<FeatherIcon color="#fff" name="shield" size={30} />
</View>
<View style={styles.alertBody}>
<Text style={styles.alertTitle}>Your account is secured!</Text>
<Text style={styles.alertMessage}>
Lorem nisi enim laboris quis elit. nisi enim laboris quis elit.
</Text>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.alertClose}>
<FeatherIcon color="#9a9a9a" name="x" size={24} />
</View>
</TouchableOpacity>
</View>
<View style={styles.alert}>
<View
style={[
styles.alertIcon,
{ backgroundColor: '#7b829b', borderColor: '#7b829b' },
]}>
<FeatherIcon color="#fff" name="video" size={30} />
</View>
<View style={styles.alertBody}>
<Text style={styles.alertTitle}>Smile! You are on camera</Text>
<Text style={styles.alertMessage}>
Lorem nisi enim laboris quis elit. nisi enim laboris quis elit.
</Text>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.alertClose}>
<FeatherIcon color="#9a9a9a" name="x" size={24} />
</View>
</TouchableOpacity>
</View>
<View style={styles.alert}>
<View
style={[
styles.alertIcon,
{ backgroundColor: '#314de7', borderColor: '#314de7' },
]}>
<FeatherIcon
color="#fff"
name="message-square"
size={30} />
</View>
<View style={styles.alertBody}>
<Text style={styles.alertTitle}>57 new messages</Text>
<Text style={styles.alertMessage}>
Lorem nisi enim laboris quis elit. nisi enim laboris quis elit.
</Text>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.alertClose}>
<FeatherIcon color="#9a9a9a" name="x" size={24} />
</View>
</TouchableOpacity>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
},
/** Alert */
alert: {
position: 'relative',
flexDirection: 'row',
marginBottom: 24,
},
alertIcon: {
padding: 16,
borderWidth: 1,
borderTopLeftRadius: 8,
borderBottomLeftRadius: 8,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
},
alertBody: {
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
padding: 16,
borderWidth: 1,
borderColor: '#ccc',
borderLeftWidth: 0,
borderTopRightRadius: 8,
borderBottomRightRadius: 8,
},
alertTitle: {
fontSize: 17,
fontWeight: '600',
color: '#1e1e1e',
marginBottom: 4,
},
alertMessage: {
fontSize: 14,
fontWeight: '500',
color: '#9c9c9c',
},
alertClose: {
position: 'absolute',
top: 0,
right: 0,
padding: 6,
alignItems: 'center',
justifyContent: 'center',
},
});
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;