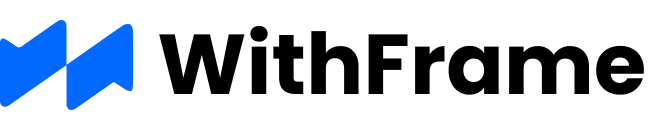
Alert: Top Banner with Dismiss Button
Screen is created using these React Native Core Components: <View />
, <SafeAreaView />
, <Text />
, <TouchableOpacity />
import React from 'react';
import {
StyleSheet,
View,
SafeAreaView,
Text,
TouchableOpacity,
} from 'react-native';
import FeatherIcon from 'react-native-vector-icons/Feather''@expo/vector-icons/Feather';
export default function Example() {
return (
<SafeAreaView>
<View style={styles.container}>
<View style={styles.alert}>
<View
style={[
styles.alertIcon,
{ backgroundColor: '#14b073', borderColor: '#14b073' },
]}>
<FeatherIcon
color="#fff"
name="credit-card"
size={30} />
</View>
<View style={styles.alertBody}>
<Text style={styles.alertTitle}>Credit card updated!</Text>
<Text style={styles.alertMessage}>
Lorem nisi enim laboris quis elit. nisi enim laboris quis elit.
</Text>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.alertClose}>
<FeatherIcon color="#9a9a9a" name="x" size={24} />
</View>
</TouchableOpacity>
</View>
<View style={styles.alert}>
<View
style={[
styles.alertIcon,
{ backgroundColor: '#fa5855', borderColor: '#fa5855' },
]}>
<FeatherIcon color="#fff" name="shield" size={30} />
</View>
<View style={styles.alertBody}>
<Text style={styles.alertTitle}>Your account is secured!</Text>
<Text style={styles.alertMessage}>
Lorem nisi enim laboris quis elit. nisi enim laboris quis elit.
</Text>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.alertClose}>
<FeatherIcon color="#9a9a9a" name="x" size={24} />
</View>
</TouchableOpacity>
</View>
<View style={styles.alert}>
<View
style={[
styles.alertIcon,
{ backgroundColor: '#7b829b', borderColor: '#7b829b' },
]}>
<FeatherIcon color="#fff" name="video" size={30} />
</View>
<View style={styles.alertBody}>
<Text style={styles.alertTitle}>Smile! You are on camera</Text>
<Text style={styles.alertMessage}>
Lorem nisi enim laboris quis elit. nisi enim laboris quis elit.
</Text>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.alertClose}>
<FeatherIcon color="#9a9a9a" name="x" size={24} />
</View>
</TouchableOpacity>
</View>
<View style={styles.alert}>
<View
style={[
styles.alertIcon,
{ backgroundColor: '#314de7', borderColor: '#314de7' },
]}>
<FeatherIcon
color="#fff"
name="message-square"
size={30} />
</View>
<View style={styles.alertBody}>
<Text style={styles.alertTitle}>57 new messages</Text>
<Text style={styles.alertMessage}>
Lorem nisi enim laboris quis elit. nisi enim laboris quis elit.
</Text>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.alertClose}>
<FeatherIcon color="#9a9a9a" name="x" size={24} />
</View>
</TouchableOpacity>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
},
/** Alert */
alert: {
position: 'relative',
flexDirection: 'row',
marginBottom: 24,
},
alertIcon: {
padding: 16,
borderWidth: 1,
borderTopLeftRadius: 8,
borderBottomLeftRadius: 8,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
},
alertBody: {
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
padding: 16,
borderWidth: 1,
borderColor: '#ccc',
borderLeftWidth: 0,
borderTopRightRadius: 8,
borderBottomRightRadius: 8,
},
alertTitle: {
fontSize: 17,
fontWeight: '600',
color: '#1e1e1e',
marginBottom: 4,
},
alertMessage: {
fontSize: 14,
fontWeight: '500',
color: '#9c9c9c',
},
alertClose: {
position: 'absolute',
top: 0,
right: 0,
padding: 6,
alignItems: 'center',
justifyContent: 'center',
},
});
Dependencies
Before getting started, make sure you have all the necessary dependencies for this component. Follow the steps below to install any missing dependencies.
-
Install the package.
npm install --save react-native-vector-icons
-
Link the native packages for iOS.
npx pod-install
In React Native 0.60+ the CLI autolink feature links the module while building the app.
-
Add Fonts to the
Info.plist
file.
Open your XCode project, right click on the Info.plist
, and select Open As -> Source Code
.
Next, copy the fonts below into your Info.plist
.
<key>UIAppFonts</key>
<array>
<string>AntDesign.ttf</string>
<string>Entypo.ttf</string>
<string>EvilIcons.ttf</string>
<string>Feather.ttf</string>
<string>FontAwesome.ttf</string>
<string>FontAwesome5_Brands.ttf</string>
<string>FontAwesome5_Regular.ttf</string>
<string>FontAwesome5_Solid.ttf</string>
<string>FontAwesome6_Brands.ttf</string>
<string>FontAwesome6_Regular.ttf</string>
<string>FontAwesome6_Solid.ttf</string>
<string>Foundation.ttf</string>
<string>Ionicons.ttf</string>
<string>MaterialIcons.ttf</string>
<string>MaterialCommunityIcons.ttf</string>
<string>SimpleLineIcons.ttf</string>
<string>Octicons.ttf</string>
<string>Zocial.ttf</string>
<string>Fontisto.ttf</string>
</array>
Note: You can only select the fonts you would like to use in your React Native Application.
After you added these fonts the Info.plist
file should look like this:
-
Add
fonts.gradle
for Android.
Open android/app/build.gradle
(NOT android/build.gradle
) and add the following code to the bottom:
apply from: file("../../node_modules/react-native-vector-icons/fonts.gradle")
If you want to customize fonts, use:
project.ext.vectoricons = [
iconFontNames: [ 'MaterialIcons.ttf', 'EvilIcons.ttf' ]
]
apply from: file("../../node_modules/react-native-vector-icons/fonts.gradle")
Original instructions can be found on Github: https://github.com/oblador/react-native-vector-icons