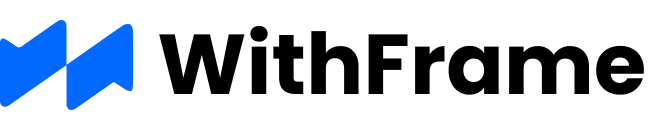
Primary Contained Button
Screen is created using these React Native Core Components: <View />
, <SafeAreaView />
, <TouchableOpacity />
, <Text />
import React from 'react';
import {
StyleSheet,
View,
SafeAreaView,
TouchableOpacity,
Text,
} from 'react-native';
export default function Example() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
<View style={styles.buttons}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnXS}>
<Text style={styles.btnXSText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnSM}>
<Text style={styles.btnSMText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnMD}>
<Text style={styles.btnMDText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnLG}>
<Text style={styles.btnLGText}>Custom Button</Text>
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnXL}>
<Text style={styles.btnXLText}>Custom Button</Text>
</View>
</TouchableOpacity>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
buttons: {
height: 300,
alignItems: 'center',
justifyContent: 'space-between',
},
btnXS: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 6,
paddingHorizontal: 14,
borderWidth: 1,
backgroundColor: '#0569FF',
borderColor: '#0569FF',
},
btnXSText: {
fontSize: 13,
lineHeight: 18,
fontWeight: '600',
color: '#fff',
},
btnSM: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#0569FF',
borderColor: '#0569FF',
},
btnSMText: {
fontSize: 14,
lineHeight: 20,
fontWeight: '600',
color: '#fff',
},
btnMD: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#0569FF',
borderColor: '#0569FF',
},
btnMDText: {
fontSize: 17,
lineHeight: 24,
fontWeight: '600',
color: '#fff',
},
btnLG: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 10,
paddingHorizontal: 20,
borderWidth: 1,
backgroundColor: '#0569FF',
borderColor: '#0569FF',
},
btnLGText: {
fontSize: 18,
lineHeight: 26,
fontWeight: '600',
color: '#fff',
},
btnXL: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 16,
paddingHorizontal: 24,
borderWidth: 1,
backgroundColor: '#0569FF',
borderColor: '#0569FF',
},
btnXLText: {
fontSize: 20,
lineHeight: 28,
fontWeight: '600',
color: '#fff',
},
});