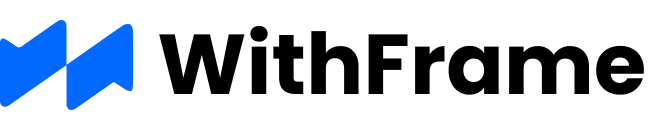
Simple Shell with Multiple Actions and Text Input
Screen is created using these React Native Core Components: <SafeAreaView />
, <View />
, <Text />
, <TouchableOpacity />
, <TextInput />
import React from 'react';
import {
StyleSheet,
SafeAreaView,
View,
Text,
TouchableOpacity,
TextInput,
} from 'react-native';
import FeatherIcon from 'react-native-vector-icons/Feather';
export default function Example() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
<View>
<View style={styles.actionWrapper}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}
style={{ marginRight: 'auto' }}>
<View style={styles.action}>
<FeatherIcon
color="#6a99e3"
name="menu"
size={22} />
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.action}>
<FeatherIcon
color="#6a99e3"
name="bell"
size={22} />
</View>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.action}>
<FeatherIcon
color="#6a99e3"
name="user"
size={22} />
</View>
</TouchableOpacity>
</View>
<Text style={styles.title}>Online Orders</Text>
<View style={styles.search}>
<View style={styles.searchInput}>
<View style={styles.inputWrapper}>
<TextInput
placeholder="Enter tracking code"
placeholderTextColor="#9eadba"
style={styles.input} />
<View style={styles.inputIcon}>
<FeatherIcon
color="#9eadba"
name="box"
size={16} />
</View>
</View>
</View>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btn}>
<Text style={styles.btnText}>Submit</Text>
</View>
</TouchableOpacity>
</View>
</View>
<View style={styles.placeholder}>
<View style={styles.placeholderInset}>
{/* Replace with your content */}
</View>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
padding: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
title: {
fontSize: 27,
fontWeight: '700',
color: '#222',
marginTop: 24,
marginBottom: 16,
},
/** Action */
action: {
width: 48,
height: 48,
borderRadius: 12,
marginHorizontal: 8,
backgroundColor: '#e8f0f9',
alignItems: 'center',
justifyContent: 'center',
},
actionWrapper: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'flex-start',
marginHorizontal: -8,
},
/** Search */
search: {
flexDirection: 'row',
alignItems: 'center',
},
searchInput: {
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
marginRight: 12,
},
/** Input */
input: {
height: 44,
backgroundColor: '#f0f6fb',
paddingLeft: 44,
paddingRight: 24,
borderRadius: 12,
fontSize: 15,
fontWeight: '500',
color: '#222',
},
inputWrapper: {
position: 'relative',
width: '100%',
},
inputIcon: {
position: 'absolute',
width: 44,
height: 44,
top: 0,
left: 0,
bottom: 0,
alignItems: 'center',
justifyContent: 'center',
},
/** Button */
btn: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 8,
paddingHorizontal: 16,
borderWidth: 1,
backgroundColor: '#222',
borderColor: '#222',
},
btnText: {
fontSize: 17,
lineHeight: 24,
fontWeight: '600',
color: '#fff',
},
/** Placeholder */
placeholder: {
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
height: 400,
marginTop: 24,
padding: 0,
backgroundColor: 'transparent',
},
placeholderInset: {
borderWidth: 4,
borderColor: '#e5e7eb',
borderStyle: 'dashed',
borderRadius: 9,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
});
Dependencies
Before getting started, make sure you have all the necessary dependencies for this component. Follow the steps below to install any missing dependencies.
-
Install the package.
npm install --save react-native-vector-icons
-
Link the native packages for iOS.
npx pod-install
In React Native 0.60+ the CLI autolink feature links the module while building the app.
-
Add Fonts to the
Info.plist
file.
Open your XCode project, right click on the Info.plist
, and select Open As -> Source Code
.
Next, copy the fonts below into your Info.plist
.
<key>UIAppFonts</key>
<array>
<string>AntDesign.ttf</string>
<string>Entypo.ttf</string>
<string>EvilIcons.ttf</string>
<string>Feather.ttf</string>
<string>FontAwesome.ttf</string>
<string>FontAwesome5_Brands.ttf</string>
<string>FontAwesome5_Regular.ttf</string>
<string>FontAwesome5_Solid.ttf</string>
<string>FontAwesome6_Brands.ttf</string>
<string>FontAwesome6_Regular.ttf</string>
<string>FontAwesome6_Solid.ttf</string>
<string>Foundation.ttf</string>
<string>Ionicons.ttf</string>
<string>MaterialIcons.ttf</string>
<string>MaterialCommunityIcons.ttf</string>
<string>SimpleLineIcons.ttf</string>
<string>Octicons.ttf</string>
<string>Zocial.ttf</string>
<string>Fontisto.ttf</string>
</array>
Note: You can only select the fonts you would like to use in your React Native Application.
After you added these fonts the Info.plist
file should look like this:
-
Add
fonts.gradle
for Android.
Open android/app/build.gradle
(NOT android/build.gradle
) and add the following code to the bottom:
apply from: file("../../node_modules/react-native-vector-icons/fonts.gradle")
If you want to customize fonts, use:
project.ext.vectoricons = [
iconFontNames: [ 'MaterialIcons.ttf', 'EvilIcons.ttf' ]
]
apply from: file("../../node_modules/react-native-vector-icons/fonts.gradle")
Original instructions can be found on Github: https://github.com/oblador/react-native-vector-icons