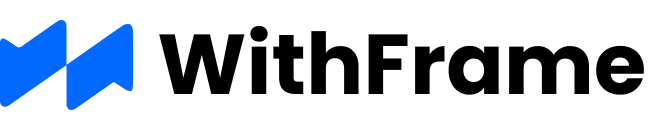
Subscription Paywall Screen with Image Background
Screen is created using these React Native Core Components: <StatusBar />
, <SafeAreaView />
, <Image />
, <View />
, <TouchableOpacity />
, <Text />
import React from 'react';
import {
StyleSheet,
Dimensions,
StatusBar,
SafeAreaView,
Image,
View,
TouchableOpacity,
Text,
} from 'react-native';
import FeatherIcon from 'react-native-vector-icons/Feather''@expo/vector-icons/Feather';
const { width, height } = Dimensions.get('window');
export default function Example() {
return (
<SafeAreaView style={{ flex: 1 }}>
<Image
alt=""
style={styles.background}
source={{
uri: 'https://images.unsplash.com/photo-1500916434205-0c77489c6cf7?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=987&q=80',
}} />
<View style={[styles.background, styles.overflow]} />
<View style={styles.container}>
<StatusBar barStyle="light-content" />
<View style={styles.paywall}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}
style={styles.paywallClose}>
<FeatherIcon color="#fff" name="x" size={30} />
</TouchableOpacity>
<Text style={styles.paywallBadge}>Limited time offer</Text>
<Text style={styles.paywallTitle}>NewsApp+</Text>
<Text style={styles.paywallMessage}>
Don't miss out on the full story. Unlock detailed reports, special
features, and more by subscribing to our premium service.
</Text>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btn}>
<Text style={styles.btnText}>Subscribe for $3.99 / mo</Text>
</View>
</TouchableOpacity>
<View style={{ marginTop: 8 }}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.btnSecondary}>
<Text style={styles.btnSecondaryText}>Skip for now</Text>
</View>
</TouchableOpacity>
</View>
</View>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
background: {
position: 'absolute',
top: 0,
left: 0,
width: width,
height: height,
},
overflow: {
backgroundColor: 'rgba(0, 0, 0, 0.6)',
},
container: {
paddingVertical: 6,
paddingHorizontal: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
/** Paywall */
paywall: {
position: 'relative',
flexDirection: 'column',
alignItems: 'stretch',
justifyContent: 'flex-end',
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
paywallClose: {
alignSelf: 'flex-end',
marginBottom: 'auto',
},
paywallBadge: {
fontSize: 15,
color: '#fff',
fontWeight: '700',
marginBottom: 4,
textAlign: 'center',
},
paywallTitle: {
fontSize: 42,
textAlign: 'center',
lineHeight: 44,
fontWeight: '700',
color: '#fff',
marginBottom: 12,
},
paywallMessage: {
textAlign: 'center',
marginBottom: 36,
fontSize: 16,
lineHeight: 22,
fontWeight: '500',
color: '#fff',
},
/** Button */
btn: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 10,
paddingHorizontal: 20,
borderWidth: 1,
backgroundColor: '#fff',
borderColor: '#fff',
},
btnText: {
fontSize: 18,
lineHeight: 26,
fontWeight: '600',
color: '#000',
},
btnSecondary: {
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderRadius: 8,
paddingVertical: 10,
paddingHorizontal: 20,
borderWidth: 1,
backgroundColor: 'transparent',
borderColor: 'transparent',
},
btnSecondaryText: {
fontSize: 18,
lineHeight: 26,
fontWeight: '600',
color: '#fff',
},
});
In-App Purchase Integration 💰
UI/UX implementation of the React Native Component with In-App Purchase integration using the `react-native-iap` package and NodeJS (Express) server.
import React from 'react';
import { StyleSheet, Text, SafeAreaView, ScrollView, StatusBar } from 'react-native';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<ScrollView style={styles.scrollView}>
<Text style={styles.text}>
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua.
</Text>
<View>
<Text style={styles.text}>in voluptate velit esse cillum dolore eu fugiat nulla</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: StatusBar.currentHeight,
},
scrollView: {
backgroundColor: 'pink',
marginHorizontal: 20,
},
text: {
fontSize: 42,
},
});
export default App;
Dependencies
Before getting started, make sure you have all the necessary dependencies for this component. Follow the steps below to install any missing dependencies.
-
Install the package.
npm install --save react-native-vector-icons
-
Link the native packages for iOS.
npx pod-install
In React Native 0.60+ the CLI autolink feature links the module while building the app.
-
Add Fonts to the
Info.plist
file.
Open your XCode project, right click on the Info.plist
, and select Open As -> Source Code
.
Next, copy the fonts below into your Info.plist
.
<key>UIAppFonts</key>
<array>
<string>AntDesign.ttf</string>
<string>Entypo.ttf</string>
<string>EvilIcons.ttf</string>
<string>Feather.ttf</string>
<string>FontAwesome.ttf</string>
<string>FontAwesome5_Brands.ttf</string>
<string>FontAwesome5_Regular.ttf</string>
<string>FontAwesome5_Solid.ttf</string>
<string>FontAwesome6_Brands.ttf</string>
<string>FontAwesome6_Regular.ttf</string>
<string>FontAwesome6_Solid.ttf</string>
<string>Foundation.ttf</string>
<string>Ionicons.ttf</string>
<string>MaterialIcons.ttf</string>
<string>MaterialCommunityIcons.ttf</string>
<string>SimpleLineIcons.ttf</string>
<string>Octicons.ttf</string>
<string>Zocial.ttf</string>
<string>Fontisto.ttf</string>
</array>
Note: You can only select the fonts you would like to use in your React Native Application.
After you added these fonts the Info.plist
file should look like this:
-
Add
fonts.gradle
for Android.
Open android/app/build.gradle
(NOT android/build.gradle
) and add the following code to the bottom:
apply from: file("../../node_modules/react-native-vector-icons/fonts.gradle")
If you want to customize fonts, use:
project.ext.vectoricons = [
iconFontNames: [ 'MaterialIcons.ttf', 'EvilIcons.ttf' ]
]
apply from: file("../../node_modules/react-native-vector-icons/fonts.gradle")
Original instructions can be found on Github: https://github.com/oblador/react-native-vector-icons