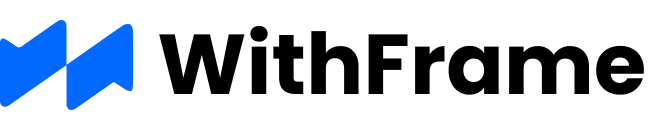
Simple Search Screen
Screen is created using these React Native Core Components: <SafeAreaView />
, <View />
, <ScrollView />
, <Text />
, <TextInput />
, <TouchableOpacity />
, <Image />
import React, { useState, useMemo } from 'react';
import {
StyleSheet,
SafeAreaView,
View,
ScrollView,
Text,
TextInput,
TouchableOpacity,
Image,
} from 'react-native';
import FeatherIcon from 'react-native-vector-icons/Feather''@expo/vector-icons/Feather';
const users = [
{
img: '',
name: 'Bell Burgess',
phone: '+1 (887) 478-2693',
},
{
img: 'https://images.unsplash.com/photo-1543610892-0b1f7e6d8ac1?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=facearea&facepad=2.5&w=256&h=256&q=80',
name: 'Bernard Baker',
phone: '+1 (862) 581-3022',
},
{
img: 'https://images.unsplash.com/photo-1494790108377-be9c29b29330?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=facearea&facepad=2.5&w=256&h=256&q=80',
name: 'Elma Chapman',
phone: '+1 (913) 497-2020',
},
{
img: 'https://images.unsplash.com/photo-1507591064344-4c6ce005b128?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=2340&q=80',
name: 'Knapp Berry',
phone: '+1 (951) 472-2967',
},
{
img: 'https://images.unsplash.com/photo-1633332755192-727a05c4013d?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=facearea&facepad=2.5&w=256&h=256&q=80',
name: 'Larson Ashbee',
phone: '+1 (972) 566-2684',
},
{
img: '',
name: 'Lorraine Abbott',
phone: '+1 (959) 422-3635',
},
{
img: 'https://images.unsplash.com/photo-1489424731084-a5d8b219a5bb?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=987&q=80',
name: 'Rosie Arterton',
phone: '+1 (845) 456-2237',
},
{
img: 'https://images.unsplash.com/photo-1573497019236-17f8177b81e8?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=2340&q=80',
name: 'Shelby Ballard',
phone: '+1 (824) 467-3579',
},
];
export default function Example() {
const [input, setInput] = useState('');
const filteredRows = useMemo(() => {
const rows = [];
const query = input.toLowerCase();
for (const item of users) {
const nameIndex = item.name.toLowerCase().search(query);
if (nameIndex !== -1) {
rows.push({
...item,
index: nameIndex,
});
}
}
return rows.sort((a, b) => a.index - b.index);
}, [input]);
return (
<SafeAreaView style={{ flex: 1, backgroundColor: '#fff' }}>
<View style={styles.container}>
<View style={styles.searchWrapper}>
<View style={styles.search}>
<View style={styles.searchIcon}>
<FeatherIcon
color="#848484"
name="search"
size={17} />
</View>
<TextInput
autoCapitalize="none"
autoCorrect={false}
clearButtonMode="while-editing"
onChangeText={val => setInput(val)}
placeholder="Start typing.."
placeholderTextColor="#848484"
returnKeyType="done"
style={styles.searchControl}
value={input} />
</View>
</View>
<ScrollView contentContainerStyle={styles.searchContent}>
{filteredRows.length ? (
filteredRows.map(({ img, name, phone }, index) => {
return (
<View key={index} style={styles.cardWrapper}>
<TouchableOpacity
onPress={() => {
// handle onPress
}}>
<View style={styles.card}>
{img ? (
<Image
alt=""
resizeMode="cover"
source={{ uri: img }}
style={styles.cardImg} />
) : (
<View style={[styles.cardImg, styles.cardAvatar]}>
<Text style={styles.cardAvatarText}>{name[0]}</Text>
</View>
)}
<View style={styles.cardBody}>
<Text style={styles.cardTitle}>{name}</Text>
<Text style={styles.cardPhone}>{phone}</Text>
</View>
<View style={styles.cardAction}>
<FeatherIcon
color="#9ca3af"
name="chevron-right"
size={22} />
</View>
</View>
</TouchableOpacity>
</View>
);
})
) : (
<Text style={styles.searchEmpty}>No results</Text>
)}
</ScrollView>
</View>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
container: {
paddingBottom: 24,
flexGrow: 1,
flexShrink: 1,
flexBasis: 0,
},
/** Search */
search: {
position: 'relative',
backgroundColor: '#efefef',
borderRadius: 12,
alignItems: 'center',
justifyContent: 'center',
flexDirection: 'row',
},
searchWrapper: {
paddingTop: 8,
paddingHorizontal: 16,
paddingBottom: 12,
borderBottomWidth: 1,
borderColor: '#efefef',
},
searchIcon: {
position: 'absolute',
top: 0,
bottom: 0,
left: 0,
width: 34,
alignItems: 'center',
justifyContent: 'center',
zIndex: 2,
},
searchControl: {
paddingVertical: 10,
paddingHorizontal: 14,
paddingLeft: 34,
width: '100%',
fontSize: 16,
fontWeight: '500',
},
searchContent: {
paddingLeft: 24,
},
searchEmpty: {
textAlign: 'center',
paddingTop: 16,
fontWeight: '500',
fontSize: 15,
color: '#9ca1ac',
},
/** Card */
card: {
paddingVertical: 14,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'flex-start',
},
cardWrapper: {
borderBottomWidth: 1,
borderColor: '#d6d6d6',
},
cardImg: {
width: 42,
height: 42,
borderRadius: 12,
},
cardAvatar: {
display: 'flex',
alignItems: 'center',
justifyContent: 'center',
backgroundColor: '#9ca1ac',
},
cardAvatarText: {
fontSize: 19,
fontWeight: 'bold',
color: '#fff',
},
cardBody: {
marginRight: 'auto',
marginLeft: 12,
},
cardTitle: {
fontSize: 16,
fontWeight: '700',
color: '#000',
},
cardPhone: {
fontSize: 15,
lineHeight: 20,
fontWeight: '500',
color: '#616d79',
marginTop: 3,
},
cardAction: {
paddingRight: 16,
},
});
Dependencies
Before getting started, make sure you have all the necessary dependencies for this component. Follow the steps below to install any missing dependencies.
-
Install the package.
npm install --save react-native-vector-icons
-
Link the native packages for iOS.
npx pod-install
In React Native 0.60+ the CLI autolink feature links the module while building the app.
-
Add Fonts to the
Info.plist
file.
Open your XCode project, right click on the Info.plist
, and select Open As -> Source Code
.
Next, copy the fonts below into your Info.plist
.
<key>UIAppFonts</key>
<array>
<string>AntDesign.ttf</string>
<string>Entypo.ttf</string>
<string>EvilIcons.ttf</string>
<string>Feather.ttf</string>
<string>FontAwesome.ttf</string>
<string>FontAwesome5_Brands.ttf</string>
<string>FontAwesome5_Regular.ttf</string>
<string>FontAwesome5_Solid.ttf</string>
<string>FontAwesome6_Brands.ttf</string>
<string>FontAwesome6_Regular.ttf</string>
<string>FontAwesome6_Solid.ttf</string>
<string>Foundation.ttf</string>
<string>Ionicons.ttf</string>
<string>MaterialIcons.ttf</string>
<string>MaterialCommunityIcons.ttf</string>
<string>SimpleLineIcons.ttf</string>
<string>Octicons.ttf</string>
<string>Zocial.ttf</string>
<string>Fontisto.ttf</string>
</array>
Note: You can only select the fonts you would like to use in your React Native Application.
After you added these fonts the Info.plist
file should look like this:
-
Add
fonts.gradle
for Android.
Open android/app/build.gradle
(NOT android/build.gradle
) and add the following code to the bottom:
apply from: file("../../node_modules/react-native-vector-icons/fonts.gradle")
If you want to customize fonts, use:
project.ext.vectoricons = [
iconFontNames: [ 'MaterialIcons.ttf', 'EvilIcons.ttf' ]
]
apply from: file("../../node_modules/react-native-vector-icons/fonts.gradle")
Original instructions can be found on Github: https://github.com/oblador/react-native-vector-icons